Terraform – Module当中使用output输出变量
output不仅仅只是在console当中输出变量,也能作为module 输出变量让其他的module使用
如何使用output在console当中输出变量?
https://www.pangzai.win/terraform-%e7%bb%93%e5%90%88%e5%a4%9a%e4%b8%aaoutput%e4%bd%bf%e7%94%a8zipmap-function%e5%88%b6%e4%bd%9c%e6%96%b0%e7%9a%84map/
以下的使用案例是
1. 使用module vpc创建 vpc,然后输出subnet id
2. 使用module ec2创建ec2 , 并且创建在这个subnet id之上
使用案例
- (vpc module)创建vpc和subnet , 输出public_subnet_id
路径: modules/vpc/main.tf
terraform {
required_version = ">= 1.0.0" # Ensure that the Terraform version is 1.0.0 or higher
required_providers {
aws = {
source = "hashicorp/aws" # Specify the source of the AWS provider
version = "~> 5.0" # Use a version of the AWS provider that is compatible with version
}
}
}
variable "environment" {}
variable "region" {}
output "public_subnet_id" {
value = aws_subnet.public_subnet_1.id
}
##############################
# Create VPC with IPv6 enabled
##############################
resource "aws_vpc" "main" {
cidr_block = "14.2.0.0/16"
assign_generated_ipv6_cidr_block = true
enable_dns_support = true
enable_dns_hostnames = true
tags = {
Name = "vpc-${var.environment}"
Environment = var.environment # Group resources by tags
}
}
##############################
# Create Internet Gateway and attach to VPC
##############################
resource "aws_internet_gateway" "igw" {
vpc_id = aws_vpc.main.id
tags = {
Name = "igw-${var.environment}"
Environment = var.environment
}
}
##############################
# Create Public Subnets with IPv6
##############################
resource "aws_subnet" "public_subnet_1" {
vpc_id = aws_vpc.main.id
cidr_block = "14.2.1.0/24"
availability_zone = "${var.region}a"
map_public_ip_on_launch = true
enable_dns64 = true
enable_resource_name_dns_aaaa_record_on_launch = true
enable_resource_name_dns_a_record_on_launch = true
# Calculate an IPv6 subnet from the VPC's IPv6 block
ipv6_cidr_block = cidrsubnet(aws_vpc.main.ipv6_cidr_block, 8, 0)
tags = {
Name = "${var.environment}-public-a"
Environment = var.environment
"karpenter.sh/discovery" = "${var.environment}-eks"
}
}
resource "aws_subnet" "public_subnet_2" {
vpc_id = aws_vpc.main.id
cidr_block = "14.2.2.0/24"
availability_zone = "${var.region}b"
map_public_ip_on_launch = true
enable_dns64 = true
enable_resource_name_dns_aaaa_record_on_launch = true
enable_resource_name_dns_a_record_on_launch = true
ipv6_cidr_block = cidrsubnet(aws_vpc.main.ipv6_cidr_block, 8, 1)
tags = {
Name = "${var.environment}-public-b"
Environment = var.environment
"karpenter.sh/discovery" = "${var.environment}-eks"
}
}
##############################
# Create Private Subnets with IPv6
##############################
resource "aws_subnet" "private_subnet_1" {
vpc_id = aws_vpc.main.id
cidr_block = "14.2.101.0/24"
availability_zone = "${var.region}a"
map_public_ip_on_launch = false
enable_dns64 = true
enable_resource_name_dns_aaaa_record_on_launch = true
enable_resource_name_dns_a_record_on_launch = true
ipv6_cidr_block = cidrsubnet(aws_vpc.main.ipv6_cidr_block, 8, 2)
tags = {
Name = "${var.environment}-private-a"
Environment = var.environment
"karpenter.sh/discovery" = "${var.environment}-eks"
}
}
resource "aws_subnet" "private_subnet_2" {
vpc_id = aws_vpc.main.id
cidr_block = "14.2.102.0/24"
availability_zone = "${var.region}b"
map_public_ip_on_launch = false
enable_dns64 = true
enable_resource_name_dns_aaaa_record_on_launch = true
enable_resource_name_dns_a_record_on_launch = true
ipv6_cidr_block = cidrsubnet(aws_vpc.main.ipv6_cidr_block, 8, 3)
tags = {
Name = "${var.environment}-private-b"
Environment = var.environment
"karpenter.sh/discovery" = "${var.environment}-eks"
}
}
##############################
# Configure Public Subnet Default Route Table and attach IGW with IPv6 route
##############################
resource "aws_route_table" "public_rt" {
#default_route_table_id = aws_vpc.main.default_route_table_id
vpc_id = aws_vpc.main.id
route {
cidr_block = "0.0.0.0/0"
gateway_id = aws_internet_gateway.igw.id
}
# Add IPv6 route
route {
ipv6_cidr_block = "::/0"
gateway_id = aws_internet_gateway.igw.id
}
tags = {
Name = "${var.environment}-public-table"
Environment = var.environment
}
}
resource "aws_route_table_association" "public_subnet_1_association" {
subnet_id = aws_subnet.public_subnet_1.id
#route_table_id = aws_default_route_table.public_rt.id
route_table_id = aws_route_table.public_rt.id
}
resource "aws_route_table_association" "public_subnet_2_association" {
subnet_id = aws_subnet.public_subnet_2.id
#route_table_id = aws_default_route_table.public_rt.id
route_table_id = aws_route_table.public_rt.id
}
##############################
# Create NAT Gateway (deployed in the Public Subnet) for outbound access from the Private Subnets
##############################
# Uncomment and configure if you want NAT Gateway for private subnets
# resource "aws_eip" "nat_eip" {
# domain = "vpc"
# }
#
# resource "aws_nat_gateway" "nat" {
# allocation_id = aws_eip.nat_eip.id
# subnet_id = aws_subnet.public_subnet_1.id
#
# tags = {
# Name = "my-nat-gateway"
# }
# }
##############################
# Create Private Subnet Route Table and attach NAT Gateway (if applicable)
##############################
resource "aws_route_table" "private_rt" {
vpc_id = aws_vpc.main.id
# For IPv4 NAT Gateway route (if NAT is enabled)
# route {
# cidr_block = "0.0.0.0/0"
# nat_gateway_id = aws_nat_gateway.nat.id
# }
tags = {
Name = "${var.environment}-private-table"
Environment = var.environment
}
}
resource "aws_route_table_association" "private_subnet_1_association" {
subnet_id = aws_subnet.private_subnet_1.id
route_table_id = aws_route_table.private_rt.id
}
resource "aws_route_table_association" "private_subnet_2_association" {
subnet_id = aws_subnet.private_subnet_2.id
route_table_id = aws_route_table.private_rt.id
}
##############################
# Create VPC Endpoint for S3 (Gateway type)
##############################
resource "aws_vpc_endpoint" "s3_endpoint" {
vpc_id = aws_vpc.main.id
service_name = "com.amazonaws.${var.region}.s3"
vpc_endpoint_type = "Gateway"
# Attach the S3 Endpoint to both Public and Private route tables
route_table_ids = [
aws_route_table.public_rt.id,
aws_route_table.private_rt.id,
]
tags = {
Name = "${var.environment}-vpc-endpoint"
Environment = var.environment
}
}
2. (ec2 module)使用vpc输出的subnet_net_id 之上创建ec2
路径: modules/ec2/main.tf
terraform {
required_version = ">= 1.0.0" # Ensure that the Terraform version is 1.0.0 or higher
required_providers {
aws = {
source = "hashicorp/aws" # Specify the source of the AWS provider
version = "~> 5.0" # Use a version of the AWS provider that is compatible with version
}
}
}
data "aws_ami" "latest_amazon_linux" {
most_recent = true
owners = ["amazon"]
filter {
name = "architecture"
values = ["x86_64"]
}
filter {
name = "name" // 找出最新版本的 Amazon Linux 2023 AMI
values = ["al2023-ami-2023*"]
}
}
resource "aws_instance" "myec2" {
ami = data.aws_ami.latest_amazon_linux.id
instance_type = var.instance_type
subnet_id = var.subnet_id
}
variable "subnet_id" {}
variable "instance_type" {}
3. 在root module当中使用
terraform {
required_version = ">= 1.0.0" # Ensure that the Terraform version is 1.0.0 or higher
required_providers {
aws = {
source = "hashicorp/aws" # Specify the source of the AWS provider
version = "~> 5.0" # Use a version of the AWS provider that is compatible with version
}
}
}
variable "region" {
default = "ap-southeast-5"
}
provider "aws" {
region = var.region
}
output "current_region" {
value = var.region
}
module "vpc" {
source = "./modules/vpc"
region = var.region
environment = "test"
}
module "ec3" {
source = "./modules/ec2"
subnet_id = module.vpc.public_subnet_id
instance_type = "t3.micro"
}
以下是结构图,ec2使用vpc当中创建的eip
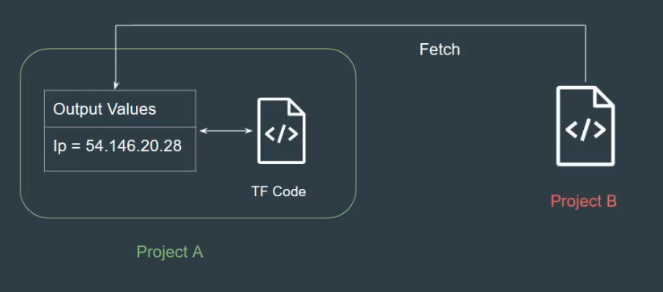
HashiCrop 推荐的module创建标准
在module当中有这些文件,那么在查看或维护也能比较容易
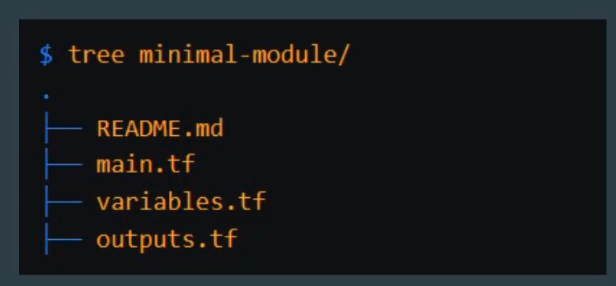
Module的服务拆分
以下是系统的要求架构图,我们可以把不同的服务都给模块化,那么下次需要使用默默服务的话就能直接使用module进行复用,而无需重头写起tf文件
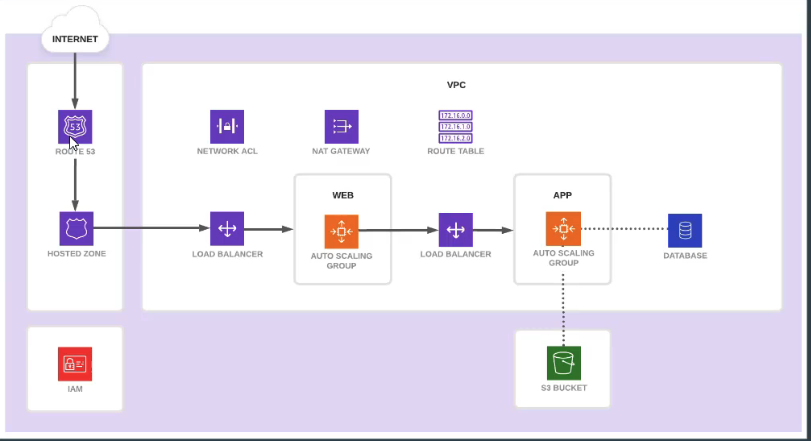
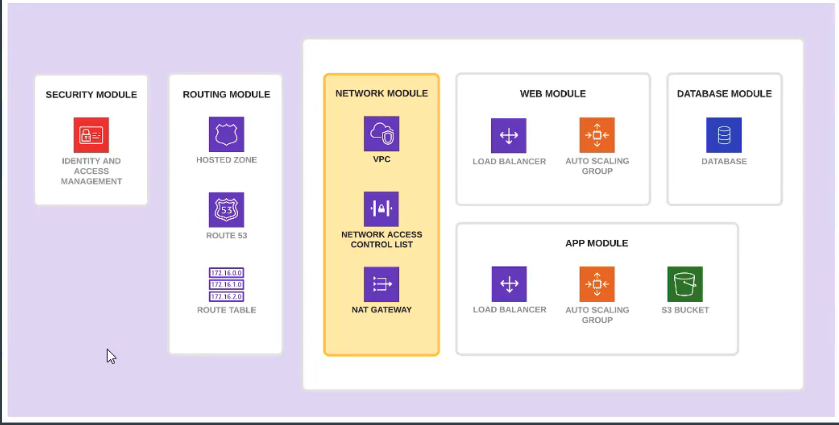
Facebook评论